Javascript Promise.All Explained
January 8, 2024
Javascript
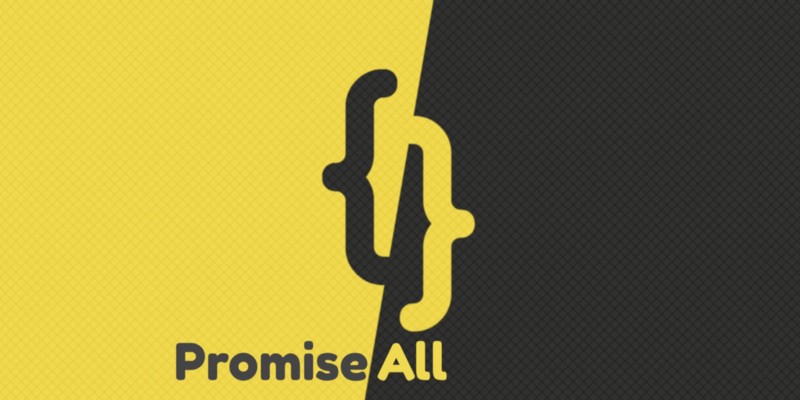
Let’s see an example with two functions that get some posts and users from the well known jsonplaceholder.typicode.com web site that has already some sample end points ready.
Here is the code:
// function1 returns a promise that resolves with posts data
async function function1() {
const response = await fetch('https://jsonplaceholder.typicode.com/posts');
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json(); // Parses and returns the JSON response
}
// function2 returns a promise that resolves with users data
async function function2() {
const response = await fetch('https://jsonplaceholder.typicode.com/users');
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json(); // Parses and returns the JSON response
}
These two functions access the network separately and each one returns a promise with some sample results.
Now since we are getting both the posts and the users that they made them, we need to wait for both sets to be downloaded, so that there are no orphaned posts or users in our forum application.
Here is how we can do that:
// Using async/await with Promise.all
async function handleFunctions() {
try {
const [posts, users] = await Promise.all([function1(), function2()]);
console.log('Posts:', posts);
console.log('Users:', users);
} catch (error) {
console.error('An error occurred:', error);
}
}
handleFunctions();